https://www.kaggle.com/competitions/2022-ai-w3p1/data
✅ 날씨정보를 이용해 배추 가격을 예측하기
(1) 배추 가격에 대한 날짜, (2) 평균기온, (3) 최저기온, (4) 최고기온, (5) 강수량 데이터가 주어질때,
배추가격을 예측하는 문제
Data Description
train.csv - 학습용 배추가격 예측을 위한 데이터
▶ year - 년,월,일 정보
▶ avgTemp - 평균기온
▶ minTemp - 최저기온
▶ maxTemp - 최고기온
▶ rainFall - 강수량
▶ avgPrice - 배추가격
test.csv - 평가용 배추가격 예측을 위한 데이터
▶ year - 년,월,일 정보
▶ avgTemp - 평균기온
▶ minTemp - 최저기온
▶ maxTemp - 최고기온
▶ rainFall - 강수량
submit_sample.csv - 평가를 위한 양식
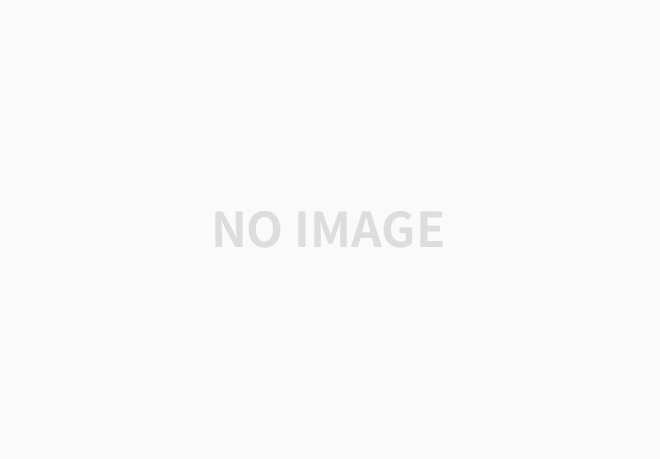
🔅 실행 후에 항상 같은 결과가 나오기 위해 random_seed 고정하기
import random
import torch
import torch.optim as optim
import torch.nn as nn
import torch.nn.functional as F
seed = 42
random.seed(seed)
np.random.seed(seed)
torch.manual_seed(seed)
if torch.cuda.is_available():
torch.cuda.manual_seed(seed)
torch.cuda.manual_seed_all(seed)
torch.backends.cudnn.benchmark = False
torch.backends.cudnn.deterministic = True
🔅 X_train, y_train 데이터셋 만들기
train = pd.read_csv('../input/2022-ai-w3p1/train.csv')
train
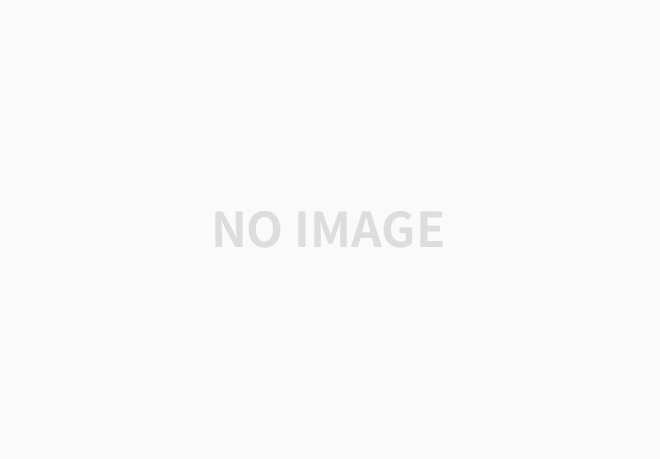
👩🏻💻 Dataframe -> numpy 배열 -> tensor로 바꾸는 과정
df.iloc: 행번호(row number)로 선택하는 방법
df.loc: label이나 조건표현으로 선택하는 방법
df.to_numpy(): Dataframe -> numpy 배열
torch.FloatTensor: numpy->tensor
X_train = train.iloc[:,:-1].to_numpy()
X_train = torch.FloatTensor(X_train)
X_train
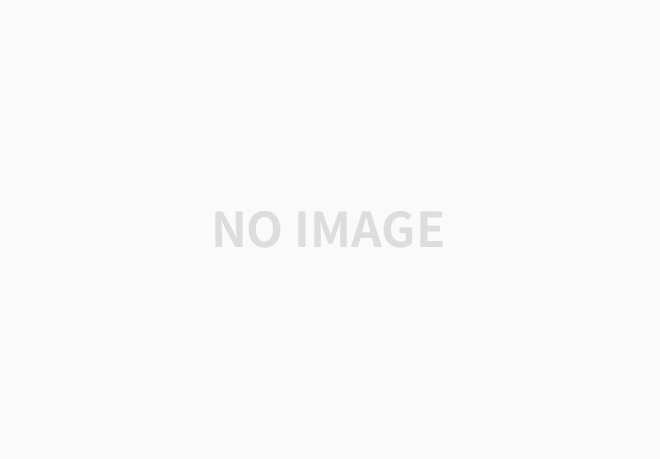
y_train = train.iloc[:,-1].to_numpy()
y_train = torch.FloatTensor(y_train)
y_train
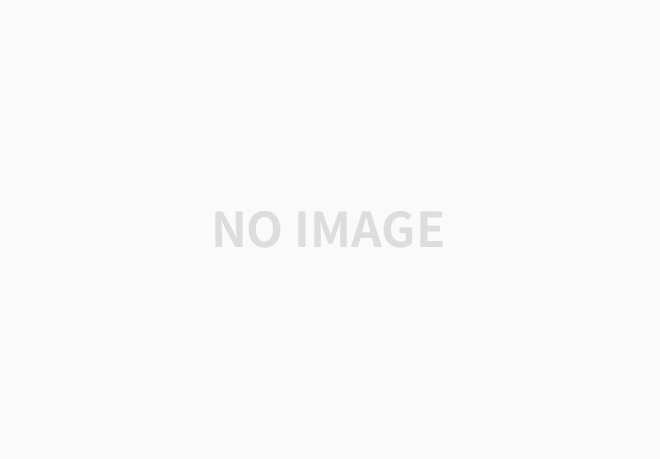
X_train, y_train 데이터셋 shape 확인하기
print(X_train.shape)
print(y_train.shape)
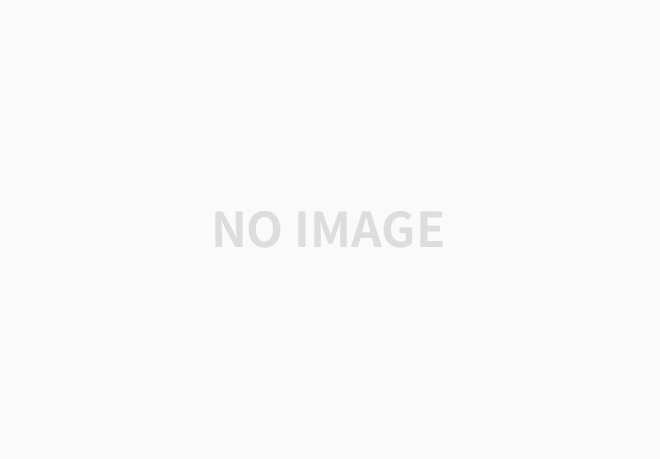
🔅 모델 훈련하기
#모델초기화
W = torch.zeros([5,1],requires_grad = True)
b = torch.zeros(1,requires_grad = True)
#optimizer설정
optimizer = optim.Adam([W,b],lr = 1e-5)
nb_epochs = 100000
for epoch in range(nb_epochs+1):
#H(x) 계산
hypothesis = X_train.matmul(W) + b
#cost 계산 (RMSE)
cost = torch.mean((hypothesis - y_train)**2)
cost = torch.sqrt(cost)
#cost로 H(x) 개선
optimizer.zero_grad()
cost.backward()
optimizer.step()
if epoch%10000 == 0:
print('Epoch {:4d}/{} hypothesis: {} Cost:{:.6f}'.format(
epoch, nb_epochs, hypothesis.squeeze().detach(), cost.item()
))
🔅 모델 테스트하기
X_test = pd.read_csv('../input/2022-ai-w3p1/test.csv').to_numpy()
X_test = torch.FloatTensor(X_test)
X_test
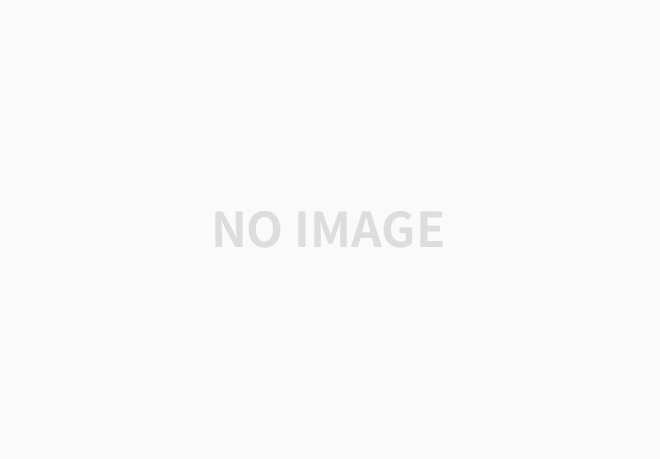
.detach(): tensor 연산 기록으로부터 분리한 tensor을 반환하는 method
tensor.numpy(): tensor -> numpy array
.squeeze(): 차원이 1인 부분을 제거
y_test = X_test.matmul(W) + b
y_test = y_test.detach().numpy().squeeze()
y_test = pd.DataFrame(y_test,columns=['Expected'])
y_test
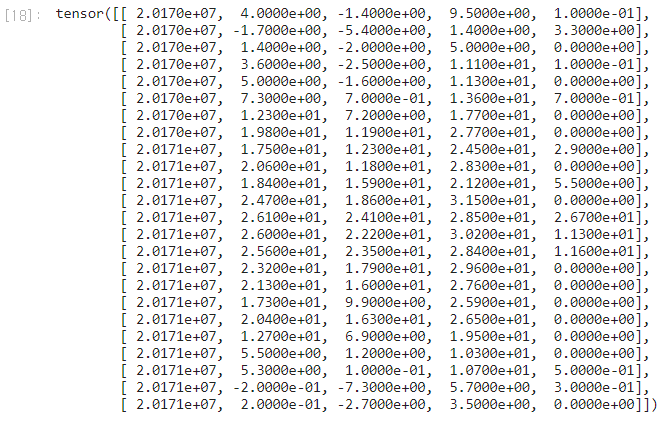
우리가 원하는 submit.csv 형태를 만들기 위해 새로운 Dataframe 만들어줌
y_test = X_test.matmul(W) + b
y_test = y_test.detach().to_numpy().squeeze()
y_test = pd.DataFrame(y_test,columns=['Expected'])
y_test
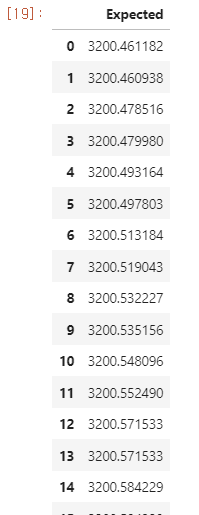
np.arange(n): 0~(n-1)인 numpy 배열 생성
Id = pd.DataFrame(np.arange(24),columns=['Id'])
Id

두 Dataframe 합치기
pd.concat([arr1,arr2],axis=): 두 Dataframe 합침
axis = 0일 때는 행으로 합치고
axis = 1일 때는 열로 합침
result = pd.concat([Id,y_test],axis=1)
result
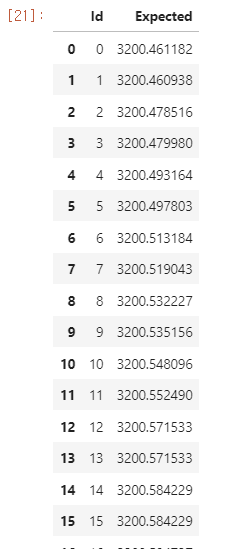
Dataframe을 csv파일로 바꾸기
df.to_csv('파일이름.csv',index=): df을 csv파일로 내보내기
index열을 만들고 싶으면 index=T, 그렇지 않으면 index=F
result.to_csv('submit.csv',index = False)
result
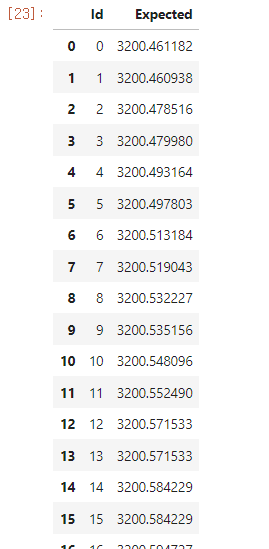
'📚 Study > AI' 카테고리의 다른 글
[딥러닝과 설계] Unsupervised Learning 비지도학습기초 (1) | 2024.07.10 |
---|---|
[cs231n] Variational Autoencoders (VAE) (0) | 2024.07.09 |
[인공지능] 3주차 | 선형회귀 실습 (0) | 2022.09.18 |
[인공지능] 3주차 | 선형회귀 개념 (0) | 2022.09.18 |
[인공지능] 2주차 | Teachable Machine 개인 프로젝트 (0) | 2022.09.18 |